Due to the fact that Ionic is a framework built atop AngularJS, like me, there may be some of you that are familiar with filters in VueJS. So to assist someone who is familiar with filters and would like to use some good old pipe-induced handlebars on-the-fly adjustments, here’s how you do it in Ionic.
First, you need to generate a Pipe. Filters are called Pipes in Ionic.
You do so with the convenient command ionic generate pipe (name)

Once generated, you need to add that pipe into the flow of your app.module.ts
file (found in your src/app folder) as follows:
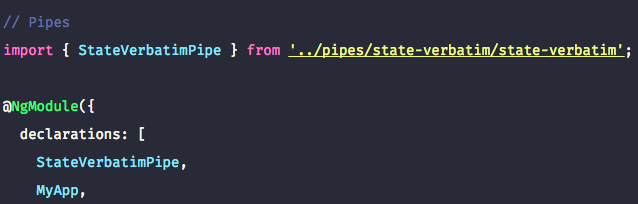
Re-run ionic lab
or ionic serve
for good measure, it’s good practice to do so when creating new integral components — think of it as a reboot.
Now you can utilise your already generated pipe as so, on a given html template: {{ 'TEXT' | stateVerbatim }}
and it should change it to all lowercase, as that is the default pipe you are supplied with:
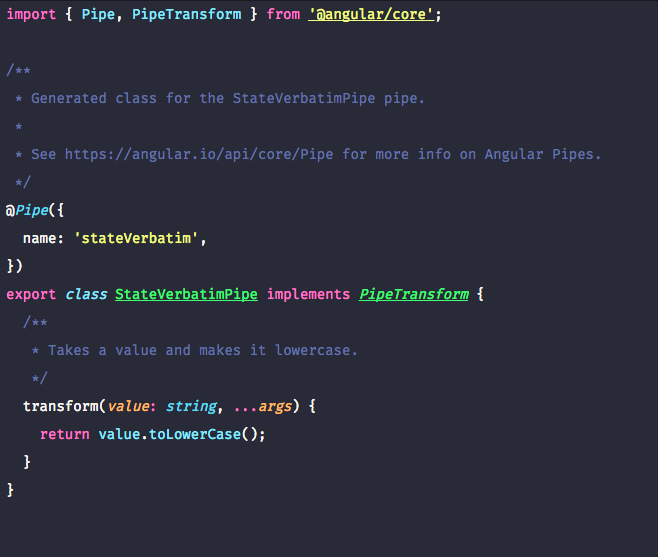
Now let’s make some small adjustments to that, so we can interact with the “value” that is being offered to the pipe’s alterations.

import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'stateVerbatim',
})
export class StateVerbatimPipe implements PipeTransform {
public states: Object = {
'WA': 'Western Australia',
'SA': 'South Australia',
'NT': 'Northern Territory',
'QLD': 'Queensland',
'TAS': 'Tasmania',
'NSW': 'New South Wales',
'VIC': 'Victoria',
'ACT': 'Australian Capital Territory'
};
transform(value: string, ...args) {
// This is our catch for data that hasn't interpolated
// from its source yet, a basic async fix.
if(value == null) return;// Otherwise, lookup the state name from the acronym
if(this.states[value]){
return this.states[value];
} else {
return value;
}
}
}
And there you have it. You now have a pipe that will turn ‘wa’ into ‘Western Australia’ and if the wa
piece of information takes a moment to arrive from an API or the backend of your app, the if(value == null) return;
part of the pipe will tell the pipe to cool its jets for a moment until the value arrives.
So, finally, usage: {{ user.state | stateVerbatim }}

Hope this makes life a bit easier for someone.